Generating and printing barcodes with using fonts is the most common way in X++ but sometimes we might need another solutions.
Finance and Operations apps include access to hundreds of standard, business-ready fonts available for documents rendered by the cloud-hosted service.
You can get more info about supported fonts in Finance and Operations here.
I want to share how to draw barcode with using fonts in X++ and save it as a bitmap or a byte array.
Below code snippet uses Barcode class and encodes the barcode with barcode type which you want to create. I choose Code128.
var barcode = Barcode::construct(BarcodeType::Code128);
barcode.string(true,"TestBarcode128");
barcode.encode();
We have the encoded barcode string in barcode.barcodeStr().
Now I am creating a class named GenerateBarcode.
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.Drawing.Text;
using System.IO;
public static class GenerateBarcode
{
}
For getting byte array of the barcode, I am adding below method.
public static System.Byte[] getByteArray(str _barcode,
str _barcodeFont, int _fontSize, int _width, int _height)
{
System.Byte[] imageBytes;
var font = new Font(_barcodeFont, _fontSize);
var rectF = new RectangleF(0, 0, _width, _height);
var image = new System.Drawing.Bitmap(_width, _height);
using (System.Drawing.Graphics graphics = System.Drawing.Graphics::FromImage(image))
{
graphics.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode::HighQualityBicubic;
graphics.TextRenderingHint = System.Drawing.Text.TextRenderingHint::ClearTypeGridFit;
graphics.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode::HighQuality;
var strFormat = System.Drawing.StringFormat::GenericTypographic;
strFormat.Alignment = System.Drawing.StringAlignment::Center;
strFormat.LineAlignment = System.Drawing.StringAlignment::Center;
var blackBrush = new SolidBrush(System.Drawing.Color::Black);
var whiteBrush = new SolidBrush(System.Drawing.Color::White);
graphics.FillRectangle(whiteBrush, rectF);
graphics.DrawString(_barcode, font, blackBrush, rectF, strFormat);
graphics.Flush();
}
using (MemoryStream ms = new MemoryStream())
{
image.Save(ms, System.Drawing.Imaging.ImageFormat::Png);
imageBytes = ms.ToArray();
}
return imageBytes;
}
For getting bitmap I'm adding this one
public static Bitmap getImage(str _barcode,
str _barcodeFont, int _fontSize, int _width, int _height)
{
var font = new Font(_barcodeFont, _fontSize);
var rectF = new RectangleF(0, 0, _width, _height);
var image = new System.Drawing.Bitmap(_width, _height);
using (System.Drawing.Graphics graphics = System.Drawing.Graphics::FromImage(image))
{
graphics.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode::HighQualityBicubic;
graphics.TextRenderingHint = System.Drawing.Text.TextRenderingHint::ClearTypeGridFit;
graphics.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode::HighQuality;
var strFormat = System.Drawing.StringFormat::GenericTypographic;
strFormat.Alignment = System.Drawing.StringAlignment::Center;
strFormat.LineAlignment = System.Drawing.StringAlignment::Center;
var blackBrush = new SolidBrush(System.Drawing.Color::Black);
var whiteBrush = new SolidBrush(System.Drawing.Color::White);
graphics.FillRectangle(whiteBrush, rectF);
graphics.DrawString(_barcode, font, blackBrush, rectF, strFormat);
graphics.Flush();
}
using (MemoryStream ms = new MemoryStream())
{
image.Save(ms, System.Drawing.Imaging.ImageFormat::Png);
}
return image;
}
Finally I can use the class in my business logic like that.
var barcode = Barcode::construct(BarcodeType::Code128);
barcode.string(true,"TestBarcode128");
barcode.encode();
var barcodeImageByteArray = GenerateBarcode::getByteArray(barcode.barcodeStr(),
"BC C128 HD Narrow",//Barcode Font
75,//Barcode Font Size
200,//Barcode Width
100//Barcode Height
);
var barcodeImage = GenerateBarcode::getImage(barcode.barcodeStr(),
"BC C128 HD Narrow",//Barcode Font
75,//Barcode Font Size
200,//Barcode Width
100//Barcode Height
);
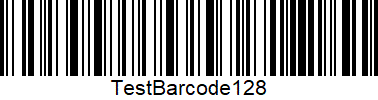
Comments